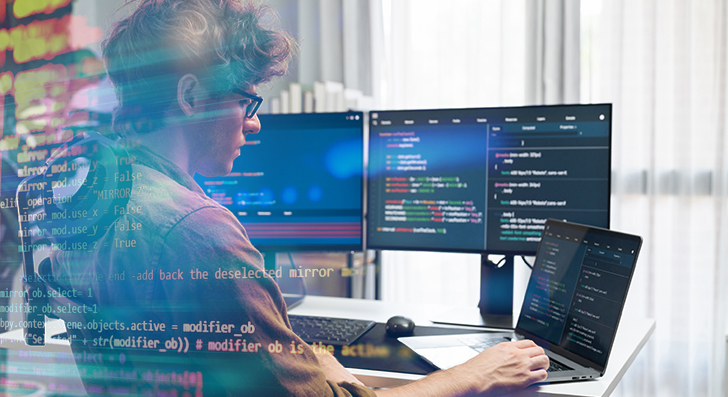
Scalability suggests your software can tackle expansion—far more customers, more facts, plus much more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your system from the beginning. Lots of programs are unsuccessful after they mature quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to think early about how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale on its own with no affecting The entire technique.
Also, give thought to your database from day one particular. Will it have to have to handle a million consumers or just a hundred? Choose the proper variety—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than current conditions. Consider what would transpire If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like information queues or occasion-driven systems. These help your application cope with additional requests devoid of finding overloaded.
If you Create with scalability in your mind, you are not just getting ready for success—you're lessening upcoming complications. A properly-planned system is less complicated to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the Right Databases
Selecting the correct databases is often a critical Section of creating scalable applications. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your application grows.
Begin by being familiar with your knowledge. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional visitors and details.
In the event your info is a lot more flexible—like consumer exercise logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing massive volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, take into account your read and compose styles. Are you undertaking many reads with less writes? Use caching and skim replicas. Are you dealing with a major create load? Investigate databases which can handle large publish throughput, or simply event-dependent data storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want Innovative scaling capabilities now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access designs. And constantly keep an eye on databases effectiveness while you increase.
Briefly, the appropriate databases is dependent upon your application’s construction, velocity demands, And just how you assume it to improve. Acquire time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is essential to scalability. As your application grows, every single modest delay adds up. Improperly published code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish efficient logic from the beginning.
Start off by composing thoroughly clean, simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most intricate Answer if a straightforward a person performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also lengthy to operate or makes use of an excessive amount of memory.
Future, have a look at your database queries. These typically slow factors down greater than the code alone. Make certain Just about every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and rather decide on specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, Specifically throughout big tables.
In case you notice the identical details becoming requested many times, use caching. Shop the outcome quickly using equipment like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app additional economical.
Make sure to examination with massive datasets. Code and queries that do the job good with one hundred information may possibly crash every time they have to take care of one million.
In short, scalable apps are quick apps. Keep your code limited, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If anything goes by just one server, it can immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server performing all of the work, the load balancer routes buyers to distinctive servers based upon availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can send visitors to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it might be reused promptly. When consumers request the exact same details again—like an item site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers database load, enhances velocity, and tends to make your application more successful.
Use caching for things which don’t modify frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they assist your app manage additional customers, remain rapid, and Recuperate from challenges. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable applications, you'll need equipment that permit your application grow effortlessly. That’s the place cloud platforms and containers can be found in. They offer you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess future capacity. When visitors raises, you'll be able to increase more resources with just a few clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are A different essential Device. A container packages your application and all the things it ought to run—code, libraries, settings—into one device. This causes it to be straightforward to move your application amongst environments, out of your notebook to your cloud, with no surprises. Docker is the most popular Software for this.
Whenever your app utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be simple to separate portions of your app into products and services. It is possible to update or scale areas independently, that is perfect for overall performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off making use of these applications early. They conserve time, lower risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location concerns early, and make greater conclusions as your application grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic may get more info help you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Regulate how much time it's going to take for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a limit or simply a company goes down, you'll want to get notified straight away. This assists you fix issues speedy, normally in advance of end users even observe.
Monitoring is also practical any time you make alterations. In case you deploy a fresh function and find out a spike in problems or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, targeted traffic and information maximize. Without checking, you’ll skip indications of problems till it’s much too late. But with the best tools set up, you remain on top of things.
In brief, checking aids you keep your app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big providers. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to Make apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create smart.